Determining Geoshell Hidden Facets [RESOLVED]
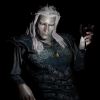
Hi All,
Does anyone know how to determine which of a GeoShel's facets are hidden and should not be exported? I couldn't find anything in the C++ docs that looks promising.
In the JSON, I found some data that seemed to match the GeoShell's options in the DAZ Studio GUI, i.e. a boolean that defines the visibility by material zone and by face group, but changing the values in DAZ Studio does not affect the values in the scene's .duf file. After I set some values to "off" in the GUI and save the scene, the values in the .duf file are all still "on", that is, true. I have no idea what is going on.
Can anyone provide a nudge in the right direction?
Thanks!
Post edited by TheMysteryIsThePoint on
Comments
Try the scripting methods added to DzGeometryShellNode in 4.16.1.37. If they do what you are looking for, you should be able to call them through a QMetaObject as I describe in this thread: Daz SDK vs Daz Script method availability
If I recall correctly, these properties are always saved with their default state (true) in the duf file, but may load disabled by editing the value to false outside of DS.
Thanks, @Omniflux
With your insights, I think I figured it out. I wrote the DAZ Script to dump some JSON describing all the visibilities. Now I'll work out calling it from C++, which I think I see how to do.
Thanks for the Christmas present :)
Hope this helps others: There doesn't appear to be a C++ exposed method or technique to do this, rather a DAZ Script API. Luckily, calling a Script from C++ is simple. I think the code @Omniflux pointed to was part of his more sophisticated asynchronous execution model. If, as in my case, a simple synchronous call is sufficient, something like this works:
QString DAZ::getGeoshellVisibilityJSON() {
static const QString source = "function getCurrentShape( node ) {\n\tvar object = node.getObject();\n\tif ( !object ) return null;\n\t\n\tvar currentShape = object.getCurrentShape();\n\tif ( !currentShape ) return null;\n\treturn currentShape;\n}\nfunction getFacetMesh( currentShape ) {\n\tvar geometry = currentShape.getGeometry();\n\tif ( !geometry ) return null;\n\tif( !geometry.inherits(\"DzFacetMesh\") ) return null;\n\treturn geometry;\n};\nfunction generateJSON( data )\n{\n // Qt-specific JSON stringifier\n function qtStringify(obj)\n {\n if (obj === null)\n {\n return \"null\";\n }\n \n if (typeof obj === \"object\")\n {\n // Handle arrays\n if (obj.constructor === Array)\n {\n var items = new Array();\n for (var i = 0; i < obj.length; ++i)\n {\n items.push(qtStringify(obj[i]));\n }\n return \"[\" + items.join(\",\") + \"]\";\n }\n // Handle objects\n else\n {\n var items = new Array();\n for (var key in obj)\n {\n if (obj.hasOwnProperty(key))\n {\n items.push('\"' + key + '\":' + qtStringify(obj[key]));\n }\n }\n return \"{\" + items.join(\",\") + \"}\";\n }\n }\n \n // Handle primitives\n if (typeof obj === \"string\")\n {\n return '\"' + obj + '\"';\n }\n \n return String(obj);\n }\n \n return qtStringify(data);\n}\nfunction modelVisibility() {\n \n var data = new Array();\n nodeCount = Scene.getNumNodes();\n for ( i = 0; i < nodeCount; i++ ) {\n var node = Scene.getNode(i);\n if ( node.inherits( \"DzGeometryShellNode\" ) ) {\n \n currentShape = getCurrentShape( node );\n if ( currentShape ) {\n var label = node.getLabel();\n fm = getFacetMesh( currentShape );\n if ( fm ) {\n var obj = new Object();\n obj.label = label;\n obj.materials = new Array();\n obj.face_groups = new Array();\n var materialsCount = currentShape.getNumMaterials();\n for (j = 0; j < materialsCount; j++ ) {\n var materialName = currentShape.getMaterial( j ).getName();\n var control = node.findMaterialGroupVisibilityControl( materialName );\n var visible = control.getValue();\n obj.materials.push( { name: materialName, value: visible });\n \n }\t\t\n var targetNode = node.getTarget();\n var targetFacetMesh = getFacetMesh( getCurrentShape( targetNode ) );\n var faceGroupCount = targetFacetMesh.getNumFaceGroups();\n for ( j = 0; j < faceGroupCount; j++ ) {\n var groupName = targetFacetMesh.getFaceGroup( j ).getName();\n var control = node.findFacetGroupVisibilityControl( groupName );\n var visible = control.getValue();\n obj.face_groups.push( { name: groupName, value: visible });\n }\n data.push( obj );\n \n }\n }\n }\n \n }\n return data;\n}\nfunction doIt() {\n var data = modelVisibility();\n var json = generateJSON( data );\n return json;\n}";
DzScript script;
script.setCode(source);
QVariantList args;
if (script.call("doIt", args)) {
auto result = script.result();
auto json = result.toString();
return json;
}
else {
throw std::runtime_error( "couldn't call geoshell visibility script" );
}
}